Factory Design Pattern
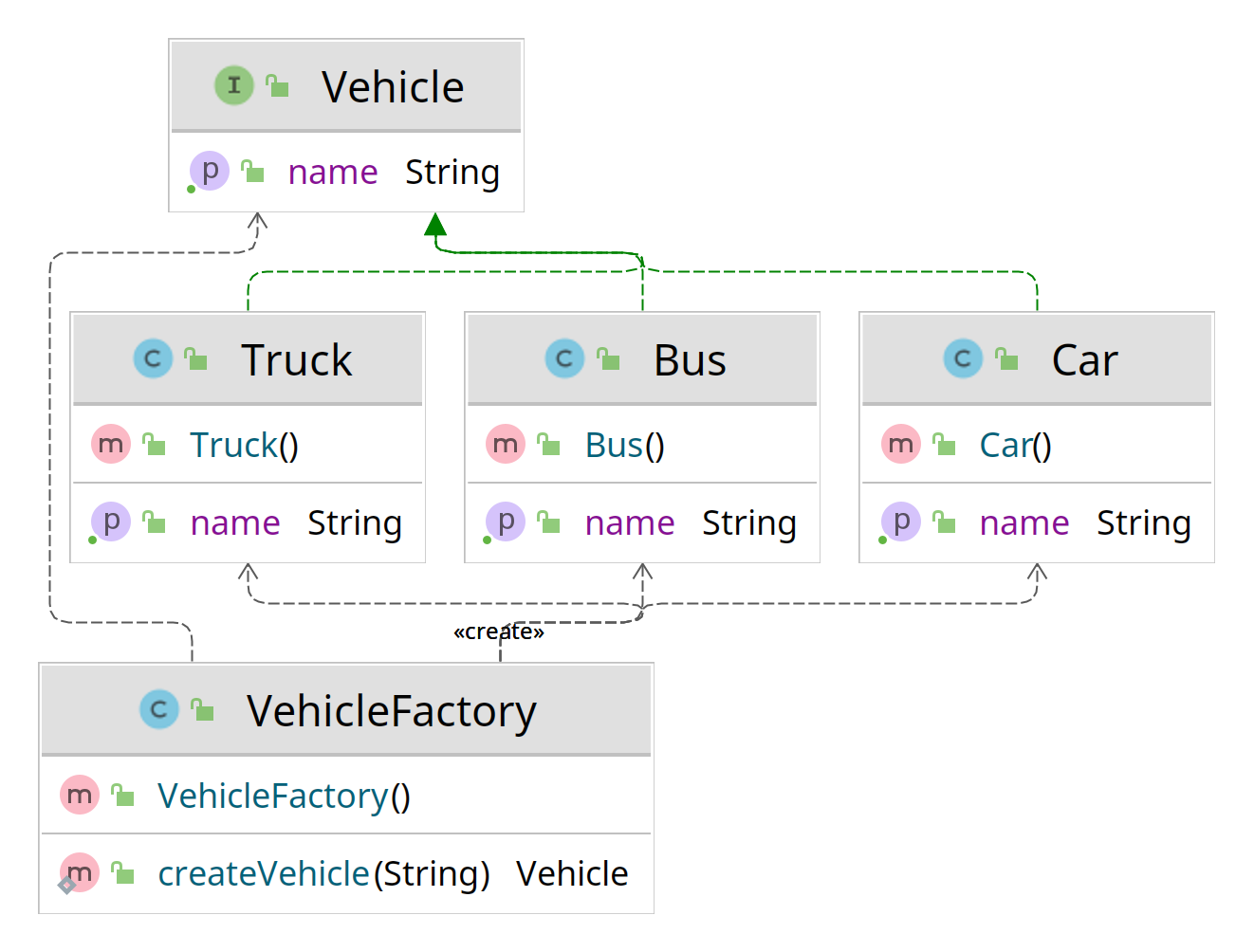
Factory design pattern is a simple and basic pattern that belongs to creational design patterns. This pattern provides an abstraction for creating objects of a specific type. To create new instances of some type, a factory is used with some arguments provided, hence the name factory pattern. Let's dive into a simple example created in Java.
First of all, we need to have an interface that specifies some basic logic our classes must implement. For that, we created a Vehicle
interface with a simple method returning a String
representing its name.
public interface Vehicle {
String getName();
}
Next we need some implementations of that Vehicle
, e.g. Car
, Truck
and Bus
.
public class Car implements Vehicle {
@Override
public String getName() {
return "Car";
}
}
public class Truck implements Vehicle {
@Override
public String getName() {
return "Truck";
}
}
public class Bus implements Vehicle {
@Override
public String getName() {
return "Bus";
}
}
Finally, let's create our factory that contains creation logic for our vehicles.
public class VehicleFactory {
public static Vehicle createVehicle(String type) {
if ("CAR".equalsIgnoreCase(type)) {
return new Car();
} else if ("TRUCK".equalsIgnoreCase(type)) {
return new Truck();
} else if ("BUS".equalsIgnoreCase(type)) {
return new Bus();
} else {
throw new IllegalArgumentException("Vehicle of type " + type + " was not recognized");
}
}
}
To create an instance of some Vehicle
, we can use createVehicle
static method inside our factory, which handles creation logic of all our vehicles. It's also abstracted as it returns a Vehicle
and not a concrete type. A sample logic is provided in the next code.
public class App {
public static void main(String[] args) {
Vehicle car = VehicleFactory.createVehicle("CAR");
// Prints: Created a vehicle of type: Car
System.out.println("Created a vehicle of type: " + car.getName());
Vehicle truck = VehicleFactory.createVehicle("TRUCK");
// Prints: Created a vehicle of type: Truck
System.out.println("Created a vehicle of type: " + truck.getName());
Vehicle bus = VehicleFactory.createVehicle("BUS");
// Prints: Created a vehicle of type: Bus
System.out.println("Created a vehicle of type: " + bus.getName());
}
}
Conclusion
That's it! We created and implemented an example of factory pattern in Java. Java standard library contains many implementations of this pattern, e.g. java.util.Calendar
, which provides four getInstance
static methods with different parameters to create an instance of a Calendar
.